CS 106 Winter 2016
Lab 08: Randomness and noise
Question 1 Warmup: random carpet
This question is a warmup: You should complete it, but you don't have to submit it. It should also be very quick.
Start with the Carpet sample sketch from Module 07. We'll change it so that the amount of recursion varies locally in a controlled but random way. The result will be a more organic and less repetitive appearance.
Right now, the decision of whether to use the base case or the recursive case in the drawCarpet() function is based purely on the current recursive level. Change the code so that once in a while, you jump into the base case even if the recursive level is not zero. Use a technique like that of the TenPrintControl sample sketch in Module 08: define a cutoff based on the mouse's current X position, and apply the base case if a random number is less than the cutoff. As a result, if you increase the number of recursive levels using the '=' key and then move the mouse left or right over the sketch, you'll get more or less of the complete fractal drawn.
Make sure that the drawing doesn't jump to a new random configuration every frame by always restarting the random number generator with the same seed before drawing the carpet.
This might sound a bit convoluted, but the changes required are pretty simple. In fact, in theory this question can be solved by adding a single line of code, and changing one existing line of code. Of course, you are welcome to make more changes if you find it to be necessary.
Name your sketch RandomCarpet. When you're done, that's it—you don't need to submit anything.
Question 2 Driving the noise function
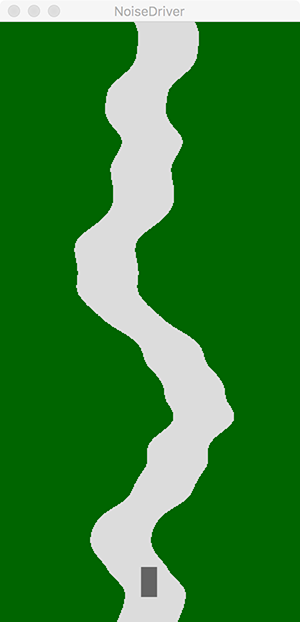
Create a simple driving game in which the road is procedurally generated using (the one-dimensional version of) the noise() function. Follow these steps:
- Create a sketch of size 300×600. In the draw() function, give it a green background.
- Now draw a horizontal one-pixel-wide light grey line in every row of pixels in the sketch. Make the line 60 pixels long. Use the one-dimensional noise() function to choose the X position of the midpoint of the line, giving it the Y coordinate of the line as input. That way, the shape of the road is fully determined by noise. The noise() function produces values between 0 and 1; map that to the largest possible range of X positions in the sketch window for which no part of the road moves off-screen.
- If you do this, you'll probably see a "road" that looks
something like this:
The problem is that you're taking values of the noise() function that are spaced too far apart, and that are therefore changing too fast. To compensate, define a global floating-point constant that acts as a scaling factor for the Y values you pass into noise(). Experiment with values of that variable until you get a road that's the right "twistiness" for a driving game. - Now let's make the road scroll down the screen. Define a global variable that controls the vertical translation of the game. Before passing Y values into the noise() function, add the current value of this global variable to them. Then, at the end of every frame, add a small amount to the translation amount. If you do this right, the road will scroll downward forever, with new segments of road always coming in from the top of the screen. Adjust the per-frame translation amount so that the road sweeps by at a reasonable rate for a driving game.
- Let's add a car to the sketch. Draw a small vertically oriented rectangle with its centre 40 pixels from the bottom of the sketch window. When the sketch starts, set the X position of the car so that it sits in the centre of the road (use a global variable to remember the car's X position). The car will always remain at this Y position in the sketch, and the road will scroll past it.
- Add the ability to control the X position of the
car via the left and right arrow keys. We want the
car to respond every frame a key is pressed without worrying
about key repeat, and so the keyPressed() hook
function isn't the best way to go. Instead, we'll use a style
of key event handling
you saw in CS 105 but haven't used this term. Add code like this
to the draw() function:
if( keyPressed ) { if( keyCode == LEFT ) { // Change the car's X position so it moves to the left. } else if( keyCode == RIGHT ) { // Change the car's X position so it moves to the right. } }
- Finally, add code to detect whether the car has gone off the road. We'll say that the car is on the road as long as the X coordinate of its centre lies somewhere within the line segment of the road at its Y position. Every frame, check whether the car has gone off the road. If it has, set a global boolean variable that stops the road from scrolling. When the road stops, you know the game is over.
The entire sketch can be written with fewer than 50 lines of code, not counting comments.
Save your sketch under the title NoiseDriver.
Submission
Remember to review the Code Style Guide and use Processing's built-in auto format tool. Then review the How To Submit document. At the top of all of your source files, be sure to include a comment with your name and student ID number. When you're ready, zip up your L08 folder, which contains the NoiseDriver sketch. (It's fine if the zip file also contains the modified Carpet sketch from Question 1, but we won't mark it.) Upload the file L08.zip to LEARN.